https://leetcode.com/problems/remove-nth-node-from-end-of-list
Given the head
of a linked list, remove the n<sup>th</sup>
node from the end of the list and return its head.
Example 1:
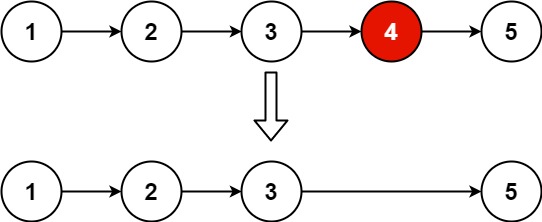
Input: head = [1,2,3,4,5], n = 2 Output: [1,2,3,5]
Example 2:
Input: head = [1], n = 1 Output: []
Example 3:
Input: head = [1,2], n = 1 Output: [1]
Constraints:
- The number of nodes in the list is
sz
. 1 <= sz <= 30
0 <= Node.val <= 100
1 <= n <= sz
Follow up: Could you do this in one pass?
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
ListNode(int x, ListNode* next) : val(x), next(next) {}
};
class Solution {
public:
ListNode* removeNthFromEnd(ListNode* head, int n) {
ListNode dummy(0, head);
ListNode* fast = &dummy;
ListNode* slow = &dummy;
// Move fast n+1 steps ahead
for (int i = 0; i <= n; i++) {
fast = fast->next;
}
// Move both pointers
while (fast) {
fast = fast->next;
slow = slow->next;
}
// Remove the nth node
slow->next = slow->next->next;
return dummy.next;
}
};
// Function to create a linked list from an array
ListNode* createLinkedList(int arr[], int size) {
if (size == 0) return nullptr;
ListNode* head = new ListNode(arr[0]);
ListNode* current = head;
for (int i = 1; i < size; i++) {
current->next = new ListNode(arr[i]);
current = current->next;
}
return head;
}
// Function to print a linked list
void printLinkedList(ListNode* head) {
while (head) {
cout << head->val << " -> ";
head = head->next;
}
cout << "NULL" << endl;
}
// Main function for testing
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
int n = 2; // Remove the 2nd node from the end
// Create linked list
ListNode* head = createLinkedList(arr, size);
cout << "Original List: ";
printLinkedList(head);
// Call removeNthFromEnd function
Solution solution;
head = solution.removeNthFromEnd(head, n);
cout << "Updated List: ";
printLinkedList(head);
return 0;
}