Given the root
of a binary tree, return the length of the diameter of the tree.
The diameter of a binary tree is the length of the longest path between any two nodes in a tree. This path may or may not pass through the root
.
The length of a path between two nodes is represented by the number of edges between them.
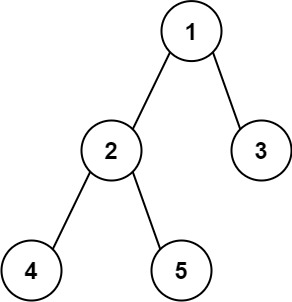
Example 1:
Input: root = [1,2,3,4,5] Output: 3
Explanation: 3 is the length of the path [4,2,1,3] or [5,2,1,3].
Example 2:
Input: root = [1,2] Output: 1
Constraints:
-100 <= Node.val <= 100
The number of nodes in the tree is in the range [1, 104].
Solution in C++ with a test case:
#include <iostream>
#include <algorithm>
using namespace std;
// Definition for a binary tree node.
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode() : val(0), left(nullptr), right(nullptr) {}
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
};
class Solution {
public:
int result;
int dfs(TreeNode* root) {
if (root == nullptr) return 0;
int left = dfs(root->left);
int right = dfs(root->right);
result = max(result, left + right);
return max(left, right) + 1;
}
int diameterOfBinaryTree(TreeNode* root) {
result = 0;
dfs(root);
return result;
}
};
// Helper function to create a tree
TreeNode* createTree() {
/*
Example tree:
1
/ \
2 3
/ \
4 5
*/
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
return root;
}
int main() {
// Create a sample binary tree
TreeNode* root = createTree();
// Create a Solution object
Solution solution;
// Call the diameterOfBinaryTree function
int diameter = solution.diameterOfBinaryTree(root);
// Output the result
cout << "Diameter of the binary tree: " << diameter << endl;
// Clean up memory (optional but good practice)
delete root->left->left;
delete root->left->right;
delete root->left;
delete root->right;
delete root;
return 0;
}